Animated Fish & Water
WebGL Maps, Water Tips
Introduction
Learn tips, with examples, to render a swimming fish with multiplied texture atlases. Generate WebGL water visual effects (VFX) with GLSL shaders. The fragment shader combines three textures representing water, a swimming fish, and rocks or gravel. This short tutorial includes each texture map, with the vertex and fragment shaders.
Texture Maps
See the fish, water, and sand texture map graphics below. Each graphic functions as a texture atlas. A texture atlas displays sections of a texture based on the current frame, allowing fast rendering of animated sprites. JavaScript increments texel values to render different areas of the graphic, per frame. Additionally the GLSL fragment shader applies these graphics to provide a unique animation.
Fish Texture Map
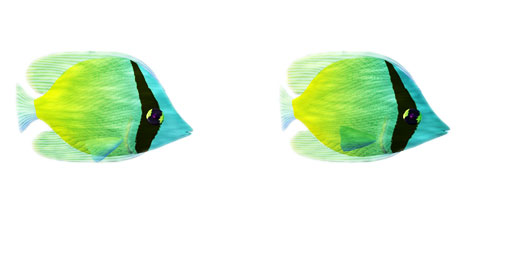
Water Texture Map
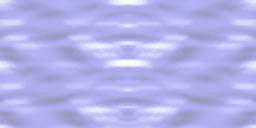
Sand Texture Map
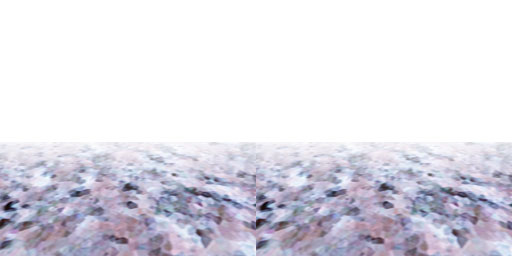
The fish was modeled with 3ds Max from photographs of a Butterfly fish.
The water graphic includes two frames with slightly different ripples of water. Water colors multiply with fish and gravel colors, in the shaders animate fish and water example, to give the appearance of moving water. Both frames of the sand graphic are identical.
Vertex Shader
The vertex shader
simply multiplies the current vertex coordinates, a_position
by the perspective matrix, um4_pmatrix
, and model matrix, um4_matrix
.
The product's output through the built in variable, gl_Position
.
The product modifies vertex coordinates per frame.
attribute vec4 a_position; attribute vec2 a_tex_coord0; varying vec2 v_tex_coord0; uniform mat4 um4_matrix; uniform mat4 um4_pmatrix; void main(void) { gl_Position = um4_pmatrix * um4_matrix * a_position; v_tex_coord0 = a_tex_coord0; }
Fragment Shader
The fragment shader samples
three different maps,
then multiplies the sampled colors together.
The built in varialble, gl_FragColor
receives the product of
the multiplication.
The final product renders to the display screen.
The sampler2D, u_sampler0
obtains color from the fish texture map.
The sampler2D, u_sampler1
samples color from the water texture map.
The sampler2D, u_sampler2
samples color from the sand texture map.
precision mediump float; uniform sampler2D u_sampler0; uniform sampler2D u_sampler1; uniform sampler2D u_sampler2; varying vec2 v_tex_coord0; void main(void) { vec4 color0 = texture2D(u_sampler0, v_tex_coord0); vec4 color1 = texture2D(u_sampler1, v_tex_coord0); vec4 color2 = texture2D(u_sampler2, v_tex_coord0); gl_FragColor = color0 * color1 * color2; }
Summary
You learned tips, with examples, to render a swimming fish with multiplied texture atlases. The fragment shader combines three textures representing water, a swimming fish, and rocks or gravel. This short tutorial included each texture map, with the vertex and fragment shaders.
Simple Shaders: Learn WebGL Book 4
Learn the basics of WebGL shader programming withSimple Shaders: Learn WebGL Book 4. This book covers seven projects with unique shaders, in detail, providing an overview of GLSL (OpenGL Shading Language), which is the shader language used for WebGL. See the following projects online
Spotlight Across Text, Radiating Colors, Cube Vertex Colors, Color Filters, Zoom Shader, Spotted Balloon Burstsand
Colors Fade. Most shaders activate as you swipe over the canvas. See various visual effects including color animation, zooming, color filters, a traveling spotlight and expanding mesh.
Simple Shaders
explains how to prepare basic WebGL GLSL shaders.
This new Kindle book includes detailed instruction and links to many interesting
WebGL shader examples online.
Learn to program WebGL shaders. Harness the power of the Graphics Processing Unit (GPU).